React
The @propelauth/react
library is designed for React frontends. This could be vanilla React managed by Vite, Next.js (either Pages or App router) with a separate backend, create-react-app, etc.
Installation
npm install @propelauth/react
Configuration
We need to tell PropelAuth where our application is running so that it will allow requests from our application. Go to the Frontend Integration section of your PropelAuth dashboard, and enter http://localhost:3000 into the Application URL field:
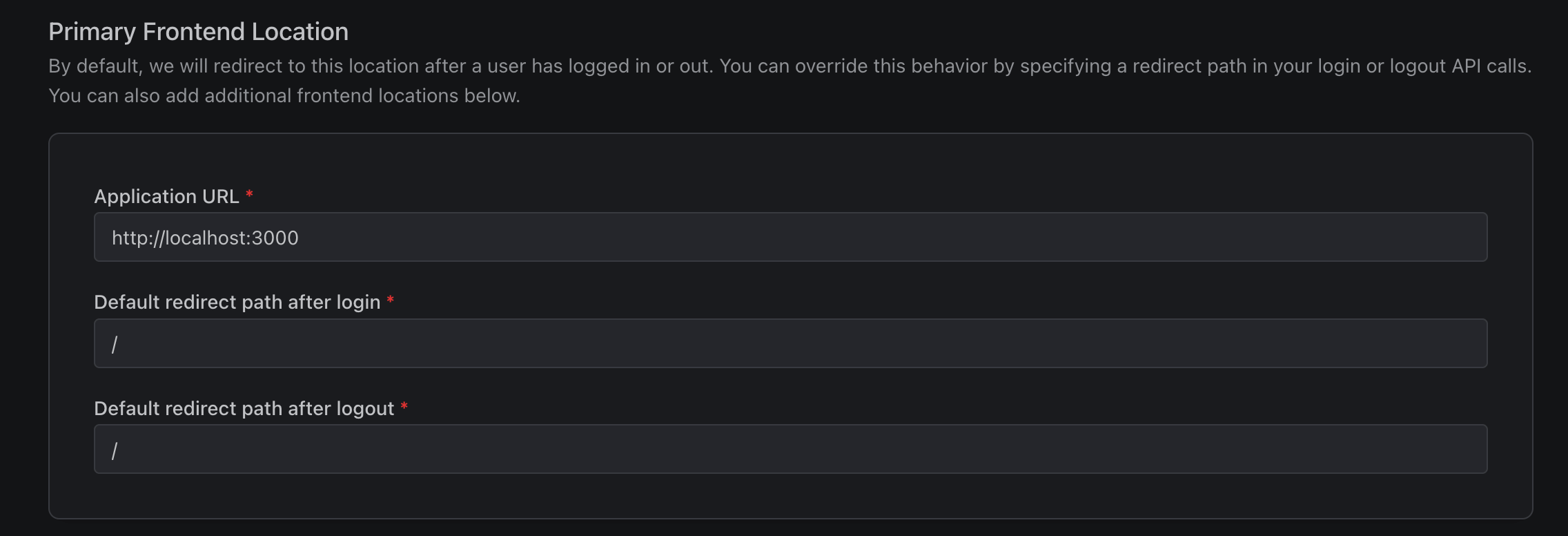
While we're here, we'll also copy the Auth URL into an .env
file, which we'll use in a second:
.env
# Test environment only, in production, we'll use our own domain
REACT_APP_AUTH_URL=https://something.propelauthtest.com
# If you are using Vite:
# VITE_AUTH_URL=https://something.propelauthtest.com
Initialization
At the root of your application, wrap your app in an AuthProvider component.
index.js
import { AuthProvider } from "@propelauth/react";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<AuthProvider authUrl={process.env.REACT_APP_AUTH_URL}>
<YourApp />
</AuthProvider>,
document.getElementById("root")
);
The AuthProvider is responsible for fetching the current user's authentication information.
If your entire application requires the user to be logged in (for example, for a dashboard),
use RequiredAuthProvider instead and you'll never have to check isLoggedIn
.
Authorization in the React library
On the frontend, authorization is useful for hiding UI elements that the user doesn't have access to. You will still need to implement authorization on the backend to prevent users from accessing data they shouldn't.
For example, you may want to hide the "Billing" page from users who aren't admins. You can do this by using the UserClass to check if the user has the "Admin" role.
const Sidebar = withRequiredAuthInfo(({userClass}) => {
// We're using the router here to get the current orgId
const router = useRouter()
const orgId = router.query.orgId as string
// Only Admins can see the billing page, so hide it from the sidebar
const isAdmin = userClass.isAtLeastRole(orgId, "Admin")
return <div>
<div>Dashboard</div>
<div>Reports</div>
{isAdmin && <div>Billing</div>}
</div>
})
AuthProvider
AuthProvider
is the provider of a
React context that manages the current
user's access token and metadata. You
cannot use the other hooks without it. AuthProvider
is the component that
manages authentication information and all other components fetches from it.
It is recommended to put the AuthProvider
component at the top level of your
application, so it never unmounts.
Properties
- Name
authUrl
- Type
- string
- Description
The base URL where your authentication pages are hosted. You can find this under the Frontend Integration section for your project.
index.js
import { AuthProvider } from "@propelauth/react";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<AuthProvider authUrl={process.env.REACT_APP_AUTH_URL}>
<YourApp />
</AuthProvider>,
document.getElementById("root")
);
RequiredAuthProvider
If your entire application requires a user to be logged in, you should prefer the RequiredAuthProvider
.
Similar to the AuthProvider, the RequiredAuthProvider manages the current user's access token and metadata.
However, the RequiredAuthProvider will also redirect the user to the login page if they are not logged in. You can override this behavior by providing a your own displayIfLoggedOut
property.
Properties
- Name
authUrl
- Type
- string
- Description
The base URL where your authentication pages are hosted. You can find this under the Frontend Integration section for your project.
- Name
displayWhileLoading
- Type
- string
- Description
A React element to display while the AuthProvider is fetching auth information. Defaults to an empty element
- Name
displayIfLoggedOut
- Type
- string
- Description
A React element to display if the current user is logged out. Defaults to <RedirectToLogin />
index.js
import { RequiredAuthProvider, RedirectToLogin } from "@propelauth/react";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<RequiredAuthProvider
authUrl={process.env.REACT_APP_AUTH_URL}
displayWhileLoading={<Loading />}
displayIfLoggedOut={<RedirectToLogin />}
>
<YourApp />
</RequiredAuthProvider>,
document.getElementById("root")
);
withAuthInfo
withAuthInfo
wraps a component and automatically adds auth information to the props of the component.
Arguments
- Name
Component
*- Type
- React.ComponentType<P extends WithAuthInfoProps>
- Description
The component to wrap
- Name
options
- Type
- WithAuthInfoArgs
- Description
Optional options for the wrapper. Currently, the only option is
displayWhileLoading
which is a React element to display while the AuthProvider is fetching auth information. Defaults to an empty element.
The following properties are added to the wrapped component's props:
Injected Properties
- Name
isLoggedIn
- Type
- boolean
- Description
Whether the user is currently logged in
- Name
accessToken
- Type
- string
- Description
The user's access token
- Name
user
- Type
- User
- Description
The user's information (e.g. email address, name, user ID). See User for all the fields on the user
- Name
userClass
- Type
- UserClass
- Description
Similar to User, but instead of just being a JSON object, this is a class with helper functions (e.g.
getOrg
,isImpersonating
). See UserClass for all the fields and helper functions.
- Name
orgHelper
- Type
- OrgHelper
- Description
A helper class for accessing the user's organization information. See OrgHelper for everything it can do.
- Name
accessHelper
- Type
- AccessHelper
- Description
A helper class for accessing the user's roles and permissions. See AccessHelper for everything it can do.
- Name
isImpersonating
- Type
- boolean
- Description
Whether the current user is being impersonated by someone else. See User Impersonation for more information.
- Name
impersonatorUserId
- Type
- string
- Description
If the current user is being impersonated, this will be the user ID of the impersonator. See User Impersonation for more information.
- Name
refreshAuthInfo
- Type
- () => Promise<void>
- Description
A function that will force refresh the current user's information. The library already refreshes this information automatically, so you should only use this if you need to force a refresh.
- Name
tokens
- Type
- Tokens
- Description
A helper class for generating access tokens. See Tokens for more information.
Signature
import {withAuthInfo} from "@propelauth/react"
withAuthInfo(Component, {
displayWhileLoading: <div>Loading...</div>
})
Example
const WelcomeMessage = withAuthInfo(props => {
if (props.isLoggedIn) {
return <div>Welcome, {props.user.email}!</div>
} else {
return <div>You aren't logged in</div>
}
})
withRequiredAuthInfo
withRequiredAuthInfo
wraps a component and automatically adds auth information to the props of the component.
It will not render the wrapped component if the user is not logged in, instead, by default, it will redirect the user to the login page.
Arguments
- Name
Component
*- Type
- React.ComponentType<P extends WithLoggedInAuthInfoProps>
- Description
The component to wrap
- Name
options
- Type
- WithRequiredAuthInfoArgs
- Description
Optional options for the wrapper. There are two options:
displayIfLoggedOut
- a React element to display if the user isn't logged in. Defaults to <RedirectToLogin />displayWhileLoading
- a React element to display while the AuthProvider is fetching auth information. Defaults to an empty element
The following properties are added to the wrapped component's props:
Injected Properties
- Name
accessToken
- Type
- string
- Description
The user's access token
- Name
user
- Type
- User
- Description
The user's information (e.g. email address, name, user ID). See User for all the fields on the user
- Name
userClass
- Type
- UserClass
- Description
Similar to User, but instead of just being a JSON object, this is a class with helper functions (e.g.
getOrg
,isImpersonating
). See UserClass for all the fields and helper functions.
- Name
orgHelper
- Type
- OrgHelper
- Description
A helper class for accessing the user's organization information. See OrgHelper for everything it can do.
- Name
accessHelper
- Type
- AccessHelper
- Description
A helper class for accessing the user's roles and permissions. See AccessHelper for everything it can do.
- Name
isImpersonating
- Type
- boolean
- Description
Whether the current user is being impersonated by someone else. See User Impersonation for more information.
- Name
impersonatorUserId
- Type
- string
- Description
If the current user is being impersonated, this will be the user ID of the impersonator. See User Impersonation for more information.
- Name
refreshAuthInfo
- Type
- () => Promise<void>
- Description
A function that will force refresh the current user's information. The library already refreshes this information automatically, so you should only use this if you need to force a refresh.
- Name
tokens
- Type
- Tokens
- Description
A helper class for generating access tokens. See Tokens for more information.
Signature
import {withRequiredAuthInfo} from "@propelauth/react"
withRequiredAuthInfo(Component, {
displayWhileLoading: <div>Loading...</div>,
displayIfLoggedOut: <RedirectToLogin />,
})
Example
const WelcomeMessage = withRequiredAuthInfo(props => {
return <div>Welcome, {props.user.email}!</div>
})
useAuthInfo
AuthInfo
is a React hook that returns the current user's information. It is similar to withAuthInfo, but instead of injecting the information into a component, it returns it from a hook.
Properties
- Name
loading
- Type
- boolean
- Description
Whether the AuthProvider is currently fetching auth information. This should only be true initially when the page loads
- Name
isLoggedIn
- Type
- boolean
- Description
Whether the user is currently logged in
- Name
accessToken
- Type
- string
- Description
The user's access token
- Name
user
- Type
- User
- Description
The user's information (e.g. email address, name, user ID). See User for all the fields on the user
- Name
userClass
- Type
- UserClass
- Description
Similar to User, but instead of just being a JSON object, this is a class with helper functions (e.g.
getOrg
,isImpersonating
). See UserClass for all the fields and helper functions.
- Name
orgHelper
- Type
- OrgHelper
- Description
A helper class for accessing the user's organization information. See OrgHelper for everything it can do.
- Name
accessHelper
- Type
- AccessHelper
- Description
A helper class for accessing the user's roles and permissions. See AccessHelper for everything it can do.
- Name
isImpersonating
- Type
- boolean
- Description
Whether the current user is being impersonated by someone else. See User Impersonation for more information.
- Name
impersonatorUserId
- Type
- string
- Description
If the current user is being impersonated, this will be the user ID of the impersonator. See User Impersonation for more information.
- Name
refreshAuthInfo
- Type
- () => Promise<void>
- Description
A function that will force refresh the current user's information. The library already refreshes this information automatically, so you should only use this if you need to force a refresh.
- Name
tokens
- Type
- Tokens
- Description
A helper class for generating access tokens. See Tokens for more information.
Signature
import {useAuthInfo} from "@propelauth/react"
const authInfo = useAuthInfo()
Example
const WelcomeMessage = () => {
const authInfo = useAuthInfo()
if (authInfo.loading) {
return <div>Loading...</div>
} else if (authInfo.isLoggedIn) {
return <div>Welcome, {authInfo.user.email}!</div>
} else {
return <div>You aren't logged in</div>
}
}
useLogoutFunction
useLogoutFunction
is a React hook that returns a function that will log the user out. It is most commonly used for creating a logout button.
The returned function takes a single argument which is whether or not to redirect the user to your pre-configured logout redirect URL. This defaults to false.
Signature
import {useLogoutFunction} from "@propelauth/react"
const logout = useLogoutFunction()
await logout(false)
Example
const LogoutButton = () => {
const logout = useLogoutFunction()
return <button onClick={() => logout(false)}>Logout</button>
}
useRedirectFunctions
useRedirectFunctions
is a React hook that returns a collection of functions which redirect the user to useful locations.
Each function takes in an optional options argument which can control features like where the user returns to after logging in.
If you need the underlying URL instead of redirecting, you can use the useHostedPageUrls hook instead.
Returned Functions
- Name
redirectToLoginPage
- Type
- (options?: RedirectToLoginOptions) => void
- Description
Redirects the user to the login page. Additional options:
- postLoginRedirectUrl - The URL to redirect the user to after they log in. Set the default in your Dashboard.
- userSignupQueryParameters - Query parameters to add to the login URL.
- Name
redirectToSignupPage
- Type
- (options?: RedirectToSignupOptions) => void
- Description
Redirects the user to the signup page. Additional options:
- postSignupRedirectUrl - The URL to redirect the user to after they sign up. Set the default in your Dashboard.
- userSignupQueryParameters - Query parameters to add to the signup URL.
- Name
redirectToAccountPage
- Type
- (options?: RedirectToAccountOptions) => void
- Description
Redirects the user to the account page. Additional options:
- redirectBackToUrl - The URL to redirect the user to when they click the back button on the account page.
- Name
redirectToOrgPage
- Type
- (orgId?: string, options?: RedirectToOrgPageOptions) => void
- Description
Redirects the user to the organization page for the given org ID. If an org ID is not provided, one is chosen automatically. Additional options:
- redirectBackToUrl - The URL to redirect the user to when they click the back button on the account page.
- Name
redirectToCreateOrgPage
- Type
- (options?: RedirectToCreateOrgOptions) => void
- Description
Redirects the user to the create organization page. Additional options:
- redirectBackToUrl - The URL to redirect the user to when they click the back button on the account page.
- Name
redirectToSetupSAMLPage
- Type
- (orgId: string, options?: RedirectToSetupSAMLPageOptions) => void
- Description
Redirects the user to a page to setup SAML for the given org ID. Additional options:
- redirectBackToUrl - The URL to redirect the user to when they click the back button on the account page.
Signature
import {useRedirectFunctions} from "@propelauth/react"
const {
redirectToLoginPage,
redirectToSignupPage,
redirectToAccountPage,
redirectToOrgPage,
redirectToCreateOrgPage,
redirectToSetupSAMLPage,
} = useRedirectFunctions()
redirectToLoginPage({
postLoginRedirectUrl: window.location.href,
userSignupQueryParameters: {
"ref": "my-cool-blog-post"
}
})
Example
const NavbarLoginButtons = withAuthInfo((props) => {
const { redirectToLoginPage, redirectToSignupPage, redirectToAccountPage } = useRedirectFunctions()
if (props.isLoggedIn) {
return <img src={props.user.pictureUrl} onClick={() => redirectToAccountPage()} />
} else {
return <div>
<button onClick={() => redirectToLoginPage()}>Login</button>
<button onClick={() => redirectToSignupPage()}>Signup</button>
</div>
}
})
useHostedPageUrls
useHostedPageUrls
is a React hook that returns a collection of functions which get URLs of useful locations.
Each function takes in an optional options argument which can control features like where the user returns to after logging in.
Returned Functions
- Name
getLoginPageUrl
- Type
- (options?: RedirectToLoginOptions) => string
- Description
Gets the URL of the login page. Additional options:
- postLoginRedirectUrl - The URL to redirect the user to after they log in. Set the default in your Dashboard.
- userSignupQueryParameters - Query parameters to add to the login URL.
- Name
getSignupPageUrl
- Type
- (options?: RedirectToSignupOptions) => string
- Description
Gets the URL of the signup page. Additional options:
- postSignupRedirectUrl - The URL to redirect the user to after they sign up. Set the default in your Dashboard.
- userSignupQueryParameters - Query parameters to add to the signup URL.
- Name
getAccountPageUrl
- Type
- (options?: RedirectToAccountOptions) => string
- Description
Gets the URL of the account page. Additional options:
- redirectBackToUrl - The URL to redirect the user to when they click the back button on the account page.
- Name
getOrgPageUrl
- Type
- (orgId?: string, options?: RedirectToOrgPageOptions) => string
- Description
Gets the URL of the organization page for the given org ID. If an org ID is not provided, one is chosen automatically. Additional options:
- redirectBackToUrl - The URL to redirect the user to when they click the back button on the account page.
- Name
getCreateOrgPageUrl
- Type
- (options?: RedirectToCreateOrgOptions) => string
- Description
Gets the URL of the create organization page. Additional options:
- redirectBackToUrl - The URL to redirect the user to when they click the back button on the account page.
- Name
getSetupSAMLPageUrl
- Type
- (orgId: string, options?: RedirectToSetupSAMLPageOptions) => string
- Description
Gets the URL of a page to setup SAML for the given org ID. Additional options:
- redirectBackToUrl - The URL to redirect the user to when they click the back button on the account page.
Signature
import {useHostedPageUrls} from "@propelauth/react"
const {
getLoginPageUrl,
getSignupPageUrl,
getAccountPageUrl,
getOrgPageUrl,
getCreateOrgPageUrl,
getSetupSAMLPageUrl,
} = useHostedPageUrls()
getLoginPageUrl({
postLoginRedirectUrl: window.location.href,
userSignupQueryParameters: {
"ref": "my-cool-blog-post"
}
})
Example
const NavbarLoginButtons = withAuthInfo((props) => {
const { getLoginPageUrl, getSignupPageUrl, getAccountPageUrl } = useHostedPageUrls()
if (props.isLoggedIn) {
return <img src={props.user.pictureUrl} onClick={() => { window.location.href = getAccountPageUrl() }} />
} else {
return <div>
<a href={getLoginPageUrl()}>Login</a>
<a href={getSignupPageUrl()}>Signup</a>
</div>
}
})
RedirectToLogin
A React component that redirects the user to the login page. It's a helpful wrapper around the useRedirectFunctions hook, specifically for login.
Properties
- Name
postLoginRedirectUrl
- Type
- string
- Description
The URL to redirect the user to after they log in. Set the default in your Dashboard.
- Name
userSignupQueryParameters
- Type
- object
- Description
Query parameters to add to the login URL.
import {RedirectToLogin} from "@propelauth/react"
<RedirectToLogin
postLoginRedirectUrl={"https://app.example.com/somewhere-else"}
userSignupQueryParameters={{
"ref": "my-cool-blog-post"
}}
/>
RedirectToSignup
A React component that redirects the user to the signup page. It's a helpful wrapper around the useRedirectFunctions hook, specifically for signup.
Properties
- Name
postSignupRedirectUrl
- Type
- string
- Description
The URL to redirect the user to after they log in. Set the default in your Dashboard.
import {RedirectToSignup} from "@propelauth/react"
<RedirectToSignup
postSignupRedirectUrl={"https://app.example.com/somewhere-else"}
/>
User
This is the object that contains all the information about the current user. It is returned from withAuthInfo, withRequiredAuthInfo, and useAuthInfo.
Properties
- Name
userId
- Type
- string
- Description
The user's unique ID
- Name
email
- Type
- string
- Description
The user's email address
- Name
createdAt
- Type
- number
- Description
The timestamp of when the user was created
- Name
firstName
- Type
- string | undefined
- Description
The user's first name
- Name
lastName
- Type
- string | undefined
- Description
The user's last name
- Name
username
- Type
- string | undefined
- Description
The user's username
- Name
properties
- Type
- object | undefined
- Description
Additional properties set on the user. See User Properties for more information.
- Name
pictureUrl
- Type
- string | undefined
- Description
The URL of the user's profile picture
- Name
hasPassword
- Type
- boolean
- Description
Whether the user has a password set. This will be false for users that signed up with a social provider (e.g. Google, Facebook), magic link, or SAML.
- Name
hasMfaEnabled
- Type
- boolean
- Description
Whether the user has 2FA authentication enabled
- Name
canCreateOrgs
- Type
- boolean
- Description
Whether the user can create organizations
- Name
legacyUserId
- Type
- string | undefined
- Description
The user's legacy user ID. This is only set if you migrated from an external source. See Migrating Users for more information.
- Name
impersonatorUserId
- Type
- string | undefined
- Description
If the current user is being impersonated, this will be the ID of the impersonator. See User Impersonation for more information.
UserClass
Similar to User, but instead of just being a JSON object, this is a class with helper functions (e.g. getOrg
, isImpersonating
).
It is returned from withAuthInfo, withRequiredAuthInfo, and useAuthInfo.
Properties
In addition to all the properties on User, this contains:
- Name
getOrg
- Type
- (orgId: string) => OrgMemberInfoClass | undefined
- Description
Returns the OrgMemberInfoClass for the given org ID
- Name
getOrgByName
- Type
- (orgName: string) => OrgMemberInfoClass | undefined
- Description
Returns the OrgMemberInfoClass for the given org name
- Name
getUserProperty
- Type
- (key: string) => unknown | undefined
- Description
Returns the value of a user property. See User Properties for more information.
- Name
getOrgs
- Type
- () => OrgMemberInfoClass[]
- Description
Returns all orgs that the user is a member of
- Name
isImpersonating
- Type
- () => boolean
- Description
Whether the current user is being impersonated by someone else. See User Impersonation for more information.
- Name
isRole
- Type
- (orgId: string, role: string) => boolean
- Description
Whether the user has the given role in the given org
- Name
isAtLeastRole
- Type
- (orgId: string, role: string) => boolean
- Description
Whether the user has at least the given role in the given org
- Name
hasPermission
- Type
- (orgId: string, permission: string) => boolean
- Description
Whether the user has the given permission in the given org
- Name
hasAllPermissions
- Type
- (orgId: string, permissions: string[]) => boolean
- Description
Whether the user has all the given permissions in the given org
Example
Example
const WelcomeMessage = withRequiredAuthInfo(({userClass}) => {
return <div>
Welcome, {userClass.firstName}!
{userClass.isImpersonating() &&
<div>You are being impersonated by {userClass.impersonatorUserId}</div>
}
</div>
})
OrgMemberInfoClass
Similar to OrgMemberInfo
, but instead of just being a JSON object, this is a class with helper functions (e.g. isRole
, hasPermission
).
It is returned from functions on the UserClass
like UserClass.getOrg and UserClass.getOrgByName.
Properties
- Name
orgId
- Type
- string
- Description
The ID of the organization
- Name
orgName
- Type
- string
- Description
The name of the organization
- Name
urlSafeOrgName
- Type
- string
- Description
The URL-safe name of the organization
- Name
orgMetadata
- Type
- object
- Description
A JSON blob of the org's metadata
- Name
userAssignedRole
- Type
- string
- Description
The role of the user within this organization
- Name
userInheritedRolesPlusCurrentRole
- Type
- string[]
- Description
The role of the user within this organization plus each inherited role
- Name
userPermissions
- Type
- string[]
- Description
The permissions of the user within this organization
- Name
isRole
- Type
- (role: string) => boolean
- Description
Whether the user has the given role in this organization
- Name
isAtLeastRole
- Type
- (role: string) => boolean
- Description
Whether the user has at least the given role in this organization
- Name
hasPermission
- Type
- (permission: string) => boolean
- Description
Whether the user has the given permission in this organization
- Name
hasAllPermissions
- Type
- (permissions: string[]) => boolean
- Description
Whether the user has all the given permissions in this organization
- Name
orgRoleStructure
- Type
- string
- Description
The role structure set for your project. See multi roles per user for more information.
- Name
userAssignedAdditionalRoles
- Type
- string[]
- Description
If using multiple roles per user, returns an array of roles that the user belongs to. Excludes the
userAssignedRole
.
- Name
legacyOrgId
- Type
- string
- Description
If the org was migrated from another system, this will be the ID of the org in the legacy system.
const org = userClass.getOrg("my-org-id")
const isAdmin = org?.isRole("Admin")
if (isAdmin) {
console.log("User is an admin in", org.orgName)
}
OrgHelper
OrgHelper
is a helper class for accessing the user's organization information. It is returned from withAuthInfo, withRequiredAuthInfo, and useAuthInfo.
You can also access it directly from useOrgHelper
if you don't need the other user information.
Signature
type OrgHelper = {
// returns all orgs that the user is a member of
getOrgs: () => OrgMemberInfo[],
// returns all org ids that the user is a member of
getOrgIds: () => string[],
// returns org information for a given orgId
getOrg: (orgId: string) => OrgMemberInfo | undefined,
// returns org information for a given org by name
getOrgByName: (orgName: string) => OrgMemberInfo | undefined,
}
type OrgMemberInfo = {
orgId: string,
orgName: string,
urlSafeOrgName: string
// The role of the user within this organization
userAssignedRole: string
userPermissions: string[]
}
Example
Org List
const OrgList = withRequiredAuthInfo(({orgHelper}) => {
return <ul>
{orgHelper.getOrgs().map(org => {
return <li key={org.orgId}>
{org.orgName}
</li>
})}
</ul>
})
AccessHelper
A helper class for accessing the user's roles and permissions. It is returned from withAuthInfo, withRequiredAuthInfo, and useAuthInfo.
Properties
- Name
isRole
- Type
- (orgId: string, role: string) => boolean
- Description
Whether the user has the given role in the given org
- Name
isAtLeastRole
- Type
- (orgId: string, role: string) => boolean
- Description
Whether the user has at least the given role in the given org
- Name
hasPermission
- Type
- (orgId: string, permission: string) => boolean
- Description
Whether the user has the given permission in the given org
- Name
hasAllPermissions
- Type
- (orgId: string, permissions: string[]) => boolean
- Description
Whether the user has all the given permissions in the given org
Example
Sidebar
const Sidebar = withRequiredAuthInfo(({accessHelper}) => {
// We're using the router here to get the current orgId
const router = useRouter()
const orgId = router.query.orgId as string
// Only Admins can see the billing page, so hide it from the sidebar
const isAdmin = accessHelper.isAtLeastRole(orgId, "Admin")
return <div>
<div>Dashboard</div>
<div>Reports</div>
{isAdmin && <div>Billing</div>}
</div>
})
Tokens
A helper class for generating access tokens.
Properties
- Name
getAccessTokenForOrg
- Type
- (orgId) => Promise<AccessTokenForActiveOrg>
- Description
A function that will return an access token that only includes the metadata for the provided org, as well as the user's metadata. Recommended for use with Active Org or to shorten the length of the access token.
- Name
getAccessToken
- Type
- () => Promise<string | undefined>
- Description
A function that will return the most up to date access token. This is great to use in components where the access token would otherwise not be updated until the component is rendered again.
Example
import {useAuthInfo} from "@propelauth/react";
const orgId = // get orgId from somewhere
async function getOrgAccessToken(orgId) {
const authInfo = useAuthInfo();
const orgAccessToken = await authInfo.tokens.getAccessTokenForOrg(orgId);
return orgAccessToken
}