Quickstart Backend Guide
In this guide, we're going to set up our backend so that it can reject unauthenticated requests from our frontend.
PropelAuth provides backend libraries for languages like Node, Python, Go, and Rust. We then also provide more specific libraries for frameworks like Express (node), FastAPI (python), Flask (python), etc.
Installation
In your FastAPI app, install the propelauth_fastapi
library.
pip install propelauth_fastapi
Initialize
You can find your Auth URL and API Key in the PropelAuth dashboard, under Backend Integration.
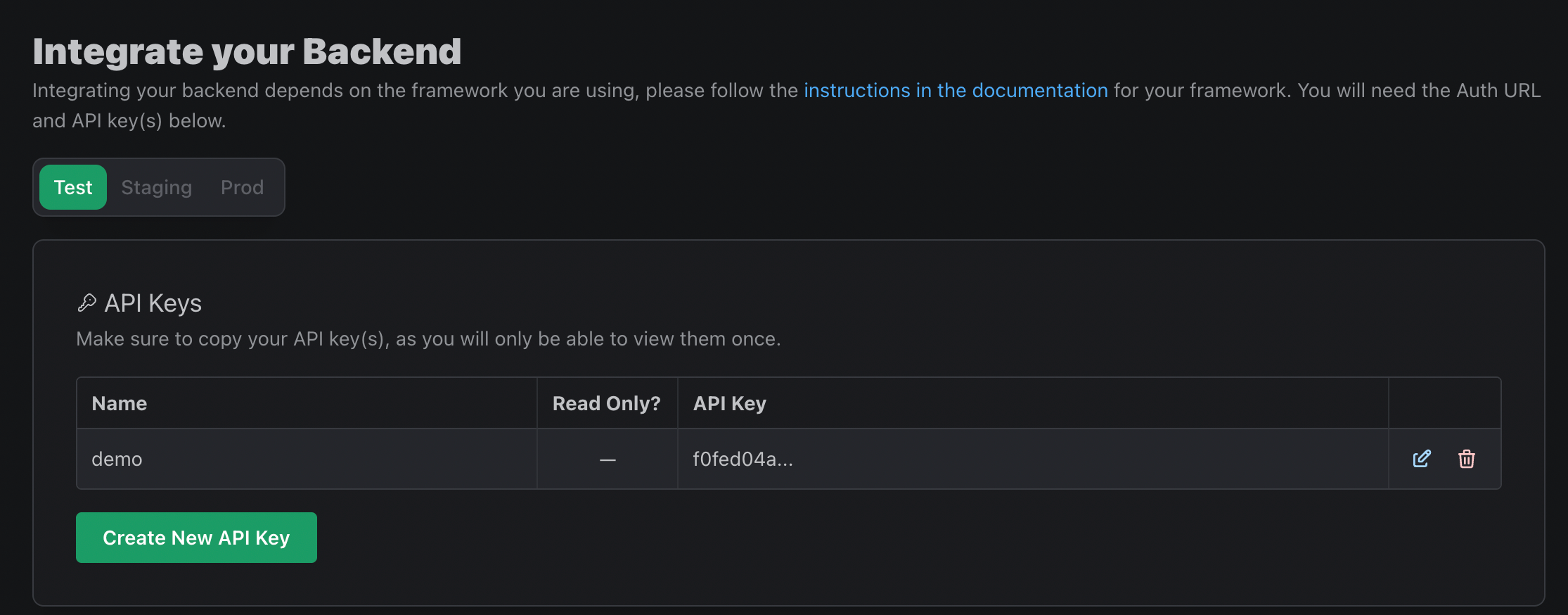
Use those two variables to initialize the library with init_auth
.
main.py
from propelauth_fastapi import init_auth
auth = init_auth("YOUR_AUTH_URL", "YOUR_API_KEY")
init_auth
performs a one-time initialization of the library.
It will verify your api_key
is correct and fetch the metadata needed to verify requests sent from the frontend.
You can optionally specify your public key to avoid a network request, which is especially useful in serverless environments.
Protecting an API Endpoint
Here is an example route can only be accessed by authenticated users:
main.py
from fastapi import Depends
from propelauth_fastapi import User
# ...
@app.get("/api/whoami")
async def root(current_user: User = Depends(auth.require_user)):
return {"user_id": f"{current_user.user_id}"}
auth.require_user will return a 401 Unauthorized for requests made without a valid access token. Requests with a valid token will have current_user set with the user’s information. The full schema is available in our reference.
You can instead use auth.optional_user if you want the request to proceed in either case.
Testing our API Without a Frontend
As the title suggests, we're going to test our API without a frontend. The frontend that we set up in the previous quickstart guide does have an easy way to get an access token, however, we're first going to look at how to test the backend in isolation.
Let's start with the simplest possible test - can unauthenticated users make requests to our API?
# Use 3001 or whichever port the backend is running on
$ curl -v localhost:3001/api/whoami
...
< HTTP/2 401
...
Great! We can see that the request was rejected with a 401 Unauthorized. To make an authenticated request, we need to pass a valid access token in the Authorization header.
You can use Create Access Token API to create a valid access token for a user. If you need the ID of a user, you can find it in the PropelAuth dashboard, under Users. Once you have an access token, you can make an authenticated request like this:
$ curl -H "Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJoaSEiOiJ0aGlzIG9uZXMgZmFrZSJ9.V712iiPYTkNqmVoISusoS7qPam7XoW8XypP4n1yd0PI"
localhost:3001/api/whoami
{"user_id":"4795fb88-0a87-4cf1-a328-8f3f9cf74497"}
And now we can see that the request was successful and the user ID was returned.
Next Steps
And now we have a backend that can reject unauthenticated requests from our frontend! But we've only made API calls from the command line. Next, we'll look at how to make authenticated requests from our frontend.